Bootstrap Tagsinput plugin is awesome. It has great functionality and lots of methods and functionalities it can offer. For more info about it, visit https://bootstrap-tagsinput.github.io/bootstrap-tagsinput/examples/
Let’s say you have this code.
<p><label for="npis">10 digit numbers</label><br />
<input type="text" id="num" name="npis" data-role="tagsinput" /><br />
<span class="v_error"> </span>
</p>
To initialize the Tagsinput plugin.
jQuery('input#num').tagsinput({
tagClass: 'badge badge-default',
maxChars: 10,
trimValue: true,
});
Now, you want to check the input first before adding them as tags, let’s say numbers only and it should be 10 digit numbers separated by commas.
// event before add tag
jQuery('input').on('beforeItemAdd', function(event) {
// event.item: contains the item
// event.cancel: set to true to prevent the item getting added
// check item if numbers only using regex
var isNum = /^\d+$/.test(event.item);
if(!isNum){
jQuery('span.v_error').html("Enter multiple numbers separated by commas (e.g., 1234567890, 4567891231)");
event.cancel = true;
} else if(event.item.length !== 10){ // check if length of input is 10
jQuery('span.v_error').html("Your numbers should be a 10-digit number.");
event.cancel = true;
} else {
jQuery('span.v_error').html('');
}
});
Now, what happens here is that, when you type in the keyboard, before the tags are created, it will detect first if there are letters or special characters in the input, if there are none and if the number input is equal to 10 digits, then the tag is created. Check the image below.
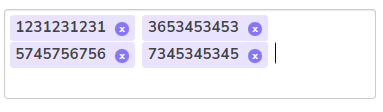
Now the problem, you want to submit these numbers to an API, then the API checks what numbers are valid or not. Let us say, NPI numbers for doctors. Each doctor has a 10-digit NPI number. So the API checks in the server database which ones are valid or not, then responds back to the website browser which ones are valid/correct or invalid/incorrect. Now we want to display it to the user by highlighting the color, valid numbers are purple/lavender, just like the image above, and invalid NPIs are orange. Also you want to be able to still type in numbers in the input to resubmit. In our case, by default, when a tag is created, I set the color to purple/lavender.
Here’s the solution.
First we need to create an empty array, this is where we will store the list of invalid numbers.
Check if there are invalid numbers by checking the length of the returned data array, then we iterate from there.
Then we use the add method from Tags Input to add the tags to the input box.
Then we push the invalid numbers to the empty array.
// create an empty array
let invalid_numbers = [];
// If number doesnt exist
// array_of_invalid_numbers will be an array of invalid numbers returned from the API
if(result_from_api.array_of_invalid_numbers.length){
for (let i = 0; i <= result_from_api.array_of_invalid_numbers.length - 1; i++){
// this is the Tagsinput method to add tags
jQuery('input#num').tagsinput('add', result_from_api.array_of_invalid_numbers[i]);
// we push the invalid numbers to the empty array invalid_numbers
invalid_numbers.push(result_from_api.array_of_invalid_numbers[i]);
}
jQuery('span.invalid_error').html("Some numbers were not found in our database. Please remove or correct the numbers highlighted in orange.");
} else {
jQuery('span.invalid_error').html('');
}
Then we get all tags added from the input box.
Then we loop through it using the jQuery function $.each().
Then we compare it to the invalid_numbers array.
Then remove or add classes.
// we get all tags by their class
// then we loop using the .each() function
// then we compare it to the invalid_numbers array
// if it matches then we remove the "badge-default" class and repalce it with "badge-invalid"
for(let inva_num = 0; inva_num < invalid_numbers.length; inva_num++){
// this is the class generated to the tag inputs elements, check it in your browser console
jQuery('.bootstrap-tagsinput span.tag').each(function(){
if(jQuery(this).text() == invalid_numbers[inva_num])
{
jQuery(this).removeClass( "badge-default" ).addClass( "badge-invalid" );
}
});
}
The final result will be like this.
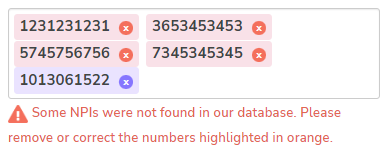
The orange ones are the invalid, and the purple one is the valid.